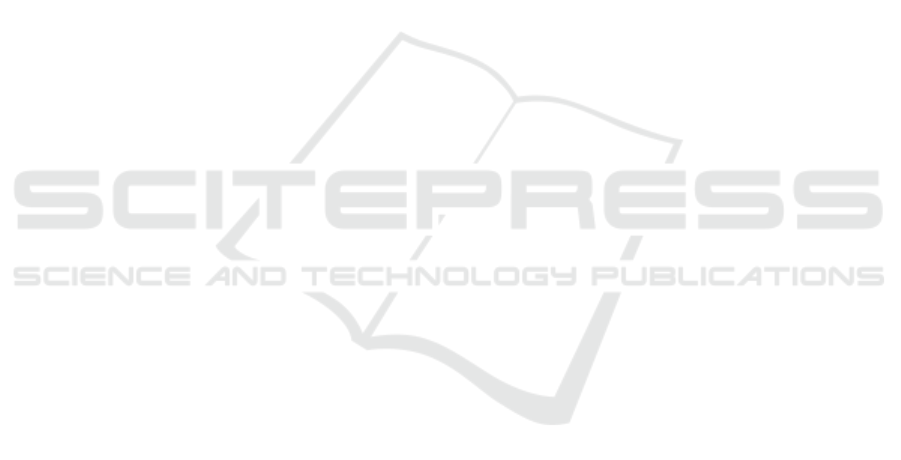
• Strategy: to easy add and interchange different al-
gorithms to solve a similar problem
• Visitor: to separate algorithms/strategies (class
Visitor) from the objects (class Element) to be ap-
plied. The methods of the classes that extends El-
ement use Double Dispatch in order to apply the
right Visitor method during execution time.
Double Dispatch is a technique that helps one to
choose what function to execute, when there exist sev-
eral with the same name, depending on the objects in-
volved in the call during the execution time.
3.2 Design
In order to build a visualization tool that can render
point clouds, polygonal and polyhedral meshes, we
have modeled the basic element by the class Element,
from which inherits the classes Point, Polygon and
Polyhedron. From Polygon inherits the class Trian-
gle and Quadrilateral, because this allows us to handle
efficiently triangular and quadrilateral meshes. From
Polyhedron inherits Tetrahedron and Hexahedron in
case of tetrahedral or hexahedral meshes are drawn.
To manage several input/output formats, render-
ing algorithms, selection modes and evaluation strate-
gies we used the Strategy pattern. For example,
we have designed the abstract class EvaluationStrat-
egy to apply any quality criterion to the individual
mesh element types. From EvaluationStrategy in-
herits MinimumAngle and MaximumDihedralAngle,
among others.
The selection strategies can be applied to the
whole mesh or to a subset of elements that was al-
ready selected. We created the class SelectionStrategy
from which inherits SelectById and SelectByProp-
erty, among others. SelectById allows a user to select
elements by using their indices and SelectByProperty
to select the elements that fulfil or not the specified
quality criterion. The Visitor pattern is implemented
between the selection strategies and the mesh ele-
ments so that by using the Double Dispatch technique
the right strategy is applied to a particular mesh ele-
ment.
The rendering strategies are also modeled in a
similar way. There exists a base class called Ren-
dererStrategy and several subclasses that implements
specific rendering algorithms. For example, Normal-
Renderer displays the model with normal vectors at
each face vertex and PropertyRenderer draws each el-
ement with different colors according to the values of
the applied quality criterion. For rendering the part
of a model that intersects 3D convex shapes, we de-
sign the class ConvexGeometryIntersectionRenderer
which inherits from RendererStrategy and Selection-
Strategy. The idea was to use the specified 3D convex
geometry for both to compute and render its intersec-
tion with the mesh and to select elements.
In order to extend the software without modifying
the user interface or already implemented classes we
have implemented dynamic registers. Each registry
class is modeled with the Singleton pattern so that
only one instance of each of them is created. Each
extensible module must have an associated registry
class. So, there exist the ModelLoadingFactory class,
the RendererRegistry class and the SelectionStrate-
gyRegistry class, among others. All these registries
inherit from a RegistryTemplate class, which stores
the different class instances associated to a key and a
priority queue that allows the programmer to organize
the order in which the different strategies will appear
in the user interface.
Finally, a Controller class, contains references to
all registry classes and is in charge of coordinating all
the operations according to the user requests.
3.3 Implementation
3.3.1 General Issues
Meshes are expected to be composed of convex poly-
gons and convex polyhedra. If non convex elements
are included, some rendering errors can appear. Since
in this application all geometrical properties remain
constant while the same model is displayed, we send
the computed values once to the GPU and keep them
in the VRAM until a new model is loaded. We man-
age attributes per triangle vertex in order to store dif-
ferent attributes when the same vertex belongs to sev-
eral triangles. So two triangles that share a vertex can
be rendered, for example, with a different flat color.
The polygons of surface meshes or the polygonal
faces of polyhedra are triangulated first before send-
ing their information to the VRAM, because GPUs
only manage triangles. Each renderer class has a con-
figuration widget, which allows the user to choose
colors and other attributes for the selected and unse-
lected elements. Each renderer has an associated Ver-
tex shader, Fragment shader and Geometry shader if
it is required. Several shaders to render the models
were taken from the book (Wolf, 2011).
In order to develop software independent of the
platform, we decided to use the Glew library (the
OpenGL Extension Wrangler library) (Glew, 2012).
This library provides efficient mechanisms to obtain
which OpenGL functionalities are available on target
GPU hardware. QtCreator was used to build the user
interface.
GRAPP 2016 - International Conference on Computer Graphics Theory and Applications
132