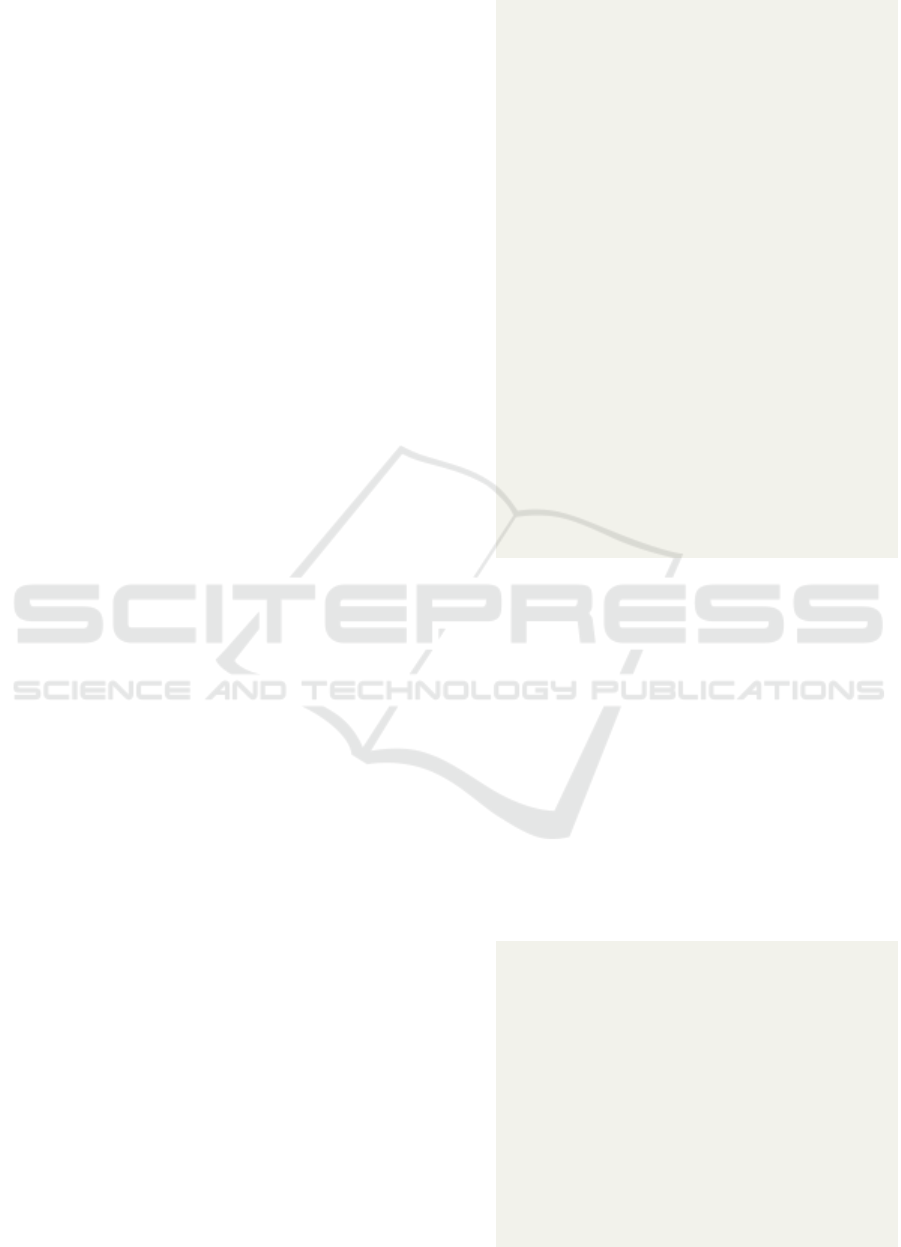
5.1 Applying JustBusiness to the
Described Scenario
To use JustBusiness, the developer needs to implement
only the business classes with their attributes, methods
and relationships. He/she should firstly identify which
business model elements are classes and which ones
are enumerations, since there is a different treatment
for each one. Classes must specialize the superclass
JBEntity, have a default constructor with no arguments,
get and set methods for each attribute, and must be
mapped with the annotations @Entity and @Table.
Enumerations should only be mapped with the anno-
tations @Enumeration and @Table. Listing 1 shows
part of the Overtime class source code. For the sake
of simplicity, some details like gets and sets, as well
as of some attributes and methods settings have been
omitted.
In line 1 the @Entity annotation is used to indicate
that Overtime class will be recognized by JustBusiness
as a business class. Using it, the developer should
inform the class label, that corresponds to the class
name, and the collectionLabel, which is equivalent to
the class plural name. In line 2, the @Table annotation
is used to create the overtime table, whose name was
informed in the name parameter, and to create the DAO
classes with the SQL queries.
After, in line 4, the @Id annotation is used to in-
dicate that the attribute id is a key in the overtime
table. The @Column annotation determines, in line 5,
that the id attribute correspond to the id_overtime col-
umn in the table. In line 6, the @Attribute annotation
specifies that an element should be recognized as an
attribute with label Identifier, has order 1, i.e., it will
be first element on screen, and will appear just in the
detail view in the user interface.
Then, in line 9, the @ManyToOne annotation spec-
ifies that an attribute represents a relationship N:1 with
the Employee class, mapped through the targetEntity
parameter. In the next line, the @JoinColumn annota-
tion informs that an attribute will perform a join opera-
tion through the id_employee with the table mapped by
the Employee class. In line 11, the @Attribute annota-
tion specifies that an element should be recognized as
an attribute with label Employee, has order 2, i.e., it
will be second element on screen, and will appear in
all views in the user interface.
Finally, the maps relating to methods approve and
pay can be seen in the lines 24 and 29, respectively.
The @Action annotation is used to identify a method
that will be available for the user. The name parameter
value is the title of the button that calls the method,
while the order parameter specifies the position in the
menu where the method appears.
1 @Entity ( l a b e l =" Ov e rt i m e " , c o l l e c t i o n L a b e l =" O v e r t i m es " )
2 @Table ( name= " o v e r t i m e " )
3 p u b l i c c l a s s Ove r t im e e x t e n d s J B E n t i t y {
4 @Id
5 @Column ( name=" i d _ o v e r t i m e " , n u l l a b l e = f a l s e , un i q u e =
t r u e )
6 @ A t t r i b u t e ( name= " I d e n t i f i e r " , o r d e r =1 , vi e w s ={ KindView
. DETAIL } )
7 I n t e g e r i d ;
8
9 @ManyToOne( t a r g e t E n t i t y =" b u s i n e s s . Emplo yee " )
10 @JoinColumn ( name= " id _ e mp l oy e e " , n u l l a b l e = f a l s e , un i q u e
= f a l s e )
11 @ A t t r i b u t e ( name= " Emplo yee " , o r d e r = 2 , v i ews ={ KindView .
ALL } )
12 Emplo yee employee ;
13
14 / / A n n o t a t i o n s o m i t t e d
15 D a t e b e g i n n i n g ;
16 D a t e e n di n g ;
17 S t r i n g d e s c r i p t i o n ;
18 S t r i n g r e mar k ;
19 S t a t u s s t a t u s = S t a t u s . WAITING_FOR_APPROVAL;
20
21 / / G e t t e r s an d S e t t e r s o m i t t e d
22 / / t o P r i m a r y D e s c r i p t i o n and t o S e c u n d a r y D e s c r i p t i o n
methods o m i t t e d
23
24 @Action ( name= " App rov e " , o r d e r =1)
25 p u b l i c v o i d a p p r ov e ( ) {
26 s t a t u s . ap p ro v e ( t h i s ) ;
27 }
28
29 @Action ( name= " Pay " , o r d e r =2 )
30 p u b l i c v o i d pay ( ) {
31 s t a t u s . pay ( t h i s ) ;
32 }
33
34 / / Ot h e r methods o m i t t e d
35 }
Listing 1: Source Code of Overtime Class.
Listing 2 shows the simplified source code of the
Employee class. Like the Overtime class, for simplic-
ity, some details like gets and sets, as well as some
attributes and methods settings have been omitted. To
avoid repetition, only the annotations that have not
been used in the the Overtime class will be explained.
In line 15, the @ManyToMany annotation specifies
that an attribute represents a relationship N:N with
the Role enumeration, mapped through the parameter
targetEntity. In the next line, the @JoinTable annota-
tion informs that the roles attribute will perform a join
operation with the table mapped by the Role enumera-
tion (shown in Listing 4) using the intermediate table
employee_role.
1 @Entity ( l a b e l =" E mp loy ee " , c o l l e c t i o n L a b e l = " E m ploye e s " )
2 @Table ( name= " em p l o y ee " )
3 p u b l i c c l a s s Emplo yee e x t e n d s J B E n t i t y {
4 @Id
5 @Column ( name=" id_ e mp l o y e e " , n u l l a b l e = f a l s e , un i q u e =
t r u e )
6 @ A t t r i b u t e ( name= " I d e n t i f i e r " , o r d e r =1 , vi e w s ={ KindView
. DETAIL } )
7 I n t e g e r i d ;
8
9 / / A n n o t a t i o n s o m i t t e d
10 S t r i n g name ;
11 S t r i n g u s e rname ;
12 S t r i n g p as s w o rd ;
13 L i s t <Overtime > o v e r t i m e s ;
14
15 @ManyToMany ( t a r g e t E n t i t y =" b u s i n e s s . Ro l e " )
16 @ J o i n Ta b l e ( name= " e m p l o y e e _ r o l e " ,
17 j o i n C o lu m n s ={ @JoinColumn ( name= " i d _e m p l o y e e " ,
18 re f e r e n c e dC o l u m nN a m e = " id _ e m p l oy e e " ) } ,
19 i n v e r s e J o i n C o l u m n s ={ @JoinColumn ( name= " i d " ,
20 re f e r e n c e dC o l u m nN a m e = " i d _ r o l e " ) } )
A Naked Objects based Framework for Developing Android Business Applications
355