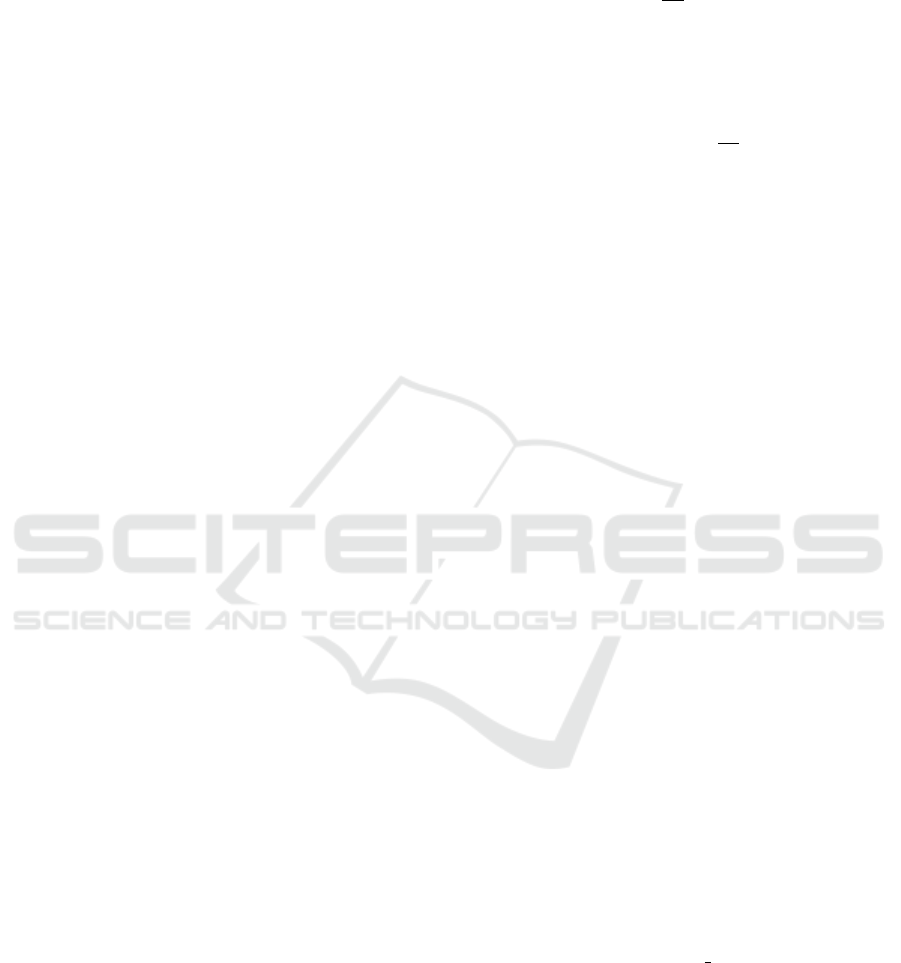
2 ALGORITHM DEVELOPMENT
AND IMPLEMENTATION
The code developed for this paper uses a mix of
Python and C++, and utilizes the Bullet physics en-
gine (Coumans, 2012). Only the BVH motion capture
file format is supported, though other formats such as
ASF/AMC could be added in the future as necessary.
The intent is to work with the large CMU Graphics
Lab Motion Capture Database and compare the orig-
inal motion captures to the generated physics anima-
tion (Calvert et al., 2003; Club, 2013). Even though
the CMU Database uses the ASF/AMC format origi-
nally, their captures have been exported to the BVH
format, and BVH is more compatible with certain
tools that sped development, such as ply, an imple-
mentation of lex and yacc in Python (Beazley, 2011;
OpenGL, 2013; SciPy, 2013; Evans, 2011). There is
no appreciable difference between the BVH files we
use here and the original ASF/AMC files, except for
the first frame which includes a T-pose of the cap-
ture’s hierarchy.
Five motion captures from the CMU motion cap-
ture database were replicated physically and had gen-
erative techniques applied.
Replicating the Motion Physically and Positional
Error. Our controllers depend on two variables: the
numerical differentiation technique and the propor-
tionality constant. We evaluated central differences
with various orders, ∇(n), where n is the order and
1 ≡ n mod 2. With each order, we also tuned the pro-
portionality constant K
f
to minimize positional error
across all joints. Because we also scale our model in
our simulation, there is a second tuning constant, K
vc
,
which scales our velocities symmetrically. That is,
v
0
( j, f ) = K
vc
v( j, f ),
for all joints j in all frames f , where v( j, f ) is joint
j’s linear velocity in frame f .
Our goal is to search for a central difference ∇(n)
and a pair (K
f
,K
vc
) that minimizes differences be-
tween the original motion capture and the simulation.
For a joint j in frame f , its positional error vector is
e
ρ
( j, f ) = r( j, f ) − ρ
ρ
ρ(r
b
( j), f ),
where ρ
ρ
ρ(r
b
( j), f ) is the position of the rigid body
r
b
( j) that corresponds to joint j in frame f , and
r( j, f ) is j’s position in the motion capture in frame
f . In an independent rigid body, ρ
ρ
ρ is the rigid body’s
center of mass, whereas for a physical skeleton, it is
at one end of the capsule shape we use for r
b
( j). The
squared error is
e
2
ρ
( j, f ) = e
ρ
( j, f ) · e
ρ
( j, f ),
which is just the dot product of e
ρ
with itself. Aver-
aging across all frames, we get
¯e
2
ρ
( j) =
1
| f |
∑
f
e
2
ρ
( j, f ),
where | f | is the number of frames. Finally, averaging
across all joints, we achieve an error number for each
pair (K
f
,K
vc
) and central difference ∇(n)
¯e
2
ρ
(K
f
,K
vc
,∇(n)) =
1
|J|
∑
j∈J
¯e
2
ρ
( j). (4)
Minimizing Equation (4) gives us tuned parame-
ters that closely match the simulation to the original
motion capture.
Search Algorithm. There are many algorithms we
could use to search for the parameters (K
f
,K
vc
,∇(n))
that minimize Equation (4). One rather simplistic ap-
proach is to generate random triples of numbers, cal-
culate Equation (4) for each triple, and compare. This
kind of search is known as a Monte Carlo search, and
is very applicable when the domain of the triples is
limited and it is difficult to obtain or apply a deter-
ministic method that optimizes these parameters. In-
deed, formulating a deterministic method appears ex-
tremely difficult, since, not only do we apply forces
and torques to each rigid body, but Bullet too applies
the force of gravity and constraint forces to each rigid
body in each frame. We therefore used a modified
Monte Carlo search. We limited our central differ-
ences to orders 3 ≤ n ≤ 19. For certain (K
f
,K
vc
),
the rigid bodies can literally fly apart in the simu-
lation, so we seed the search initially with a known
good pair, (K
g
f
,K
g
vc
). Algorithm 1 details the search
method, where random[−1, 1] is a function that pro-
duces a random real number, i.e., a floating point
number, between -1 and 1 inclusive. It can easily be
seen that this algorithm runs in O(points · maxOrder ·
F(mocap-file)), where F is the number of frames in
mocap-file.
We found that a good starting point for indepen-
dent rigid bodies was (K
g
f
,K
g
vc
) = (100, 75), and for
physical skeletons was (K
g
F
,K
g
vc
) = (20,100). These
parameters produced a simulation that at least some-
what matched all of the captures. We then tuned to
a single motion capture, 141 15.bvh. This capture is
by far the longest of our 5, and demonstrates many
interactions between joints by going through ranges
of motion. From there, we used 100 points per run,
each run taking about 14 minutes on a laptop, and a
maximum order of 19. We then used the best tun-
ing parameters from each run to seed the good values
of the subsequent run. A concern with this particular
method is that runs will not converge upon a better
solution. However, we found that, starting with the
Generative Animation in a Physics Engine using Motion Captures
253