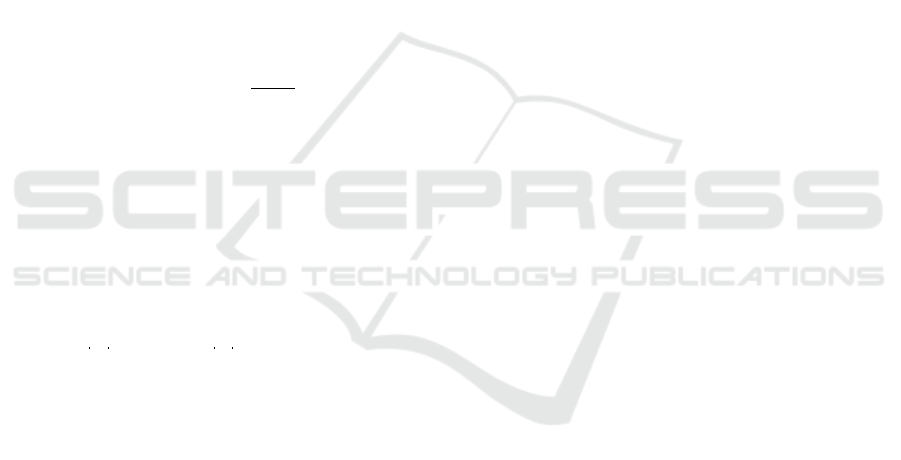
1. First, we compute the coefficients of the binomial
expansion of (1−r)
l
using Pascal’s Triangle. The
coefficients are stored in an array (a vector) whose
indexing corresponds to the degree of the respec-
tive polynomial term. In more detail, at the n-th
position we store the coefficient of the term r
n
for
n = 0,. . . , l. The dimension of the storage in this
part of the code will be l + 1.
2. Once the binomial expansion is computed, the
first multiplication t(1 − t)
l
must be performed.
This multiplication will only increase each term’s
degree by one, without affecting the coefficients,
hence, we move the coefficients from the previous
iteration into the next position in the array. So,
the coefficient of t
n
will become the coefficient of
t
n+1
for n = 0,...,l.
3. The integration will also increase the length of the
array by one. The difference is that in this case
the coefficients will be integrated using the simple
formula (without the integration constant):
Z
at
n
dt =
at
n+1
n + 1
Therefore, the coefficient of t
n
will be divided by
n + 1 and stored in the position n + 1 of the new
array.
4. The limits of the integration are r and 1. Evaluat-
ing a polynomial at 1 is achieved by adding up all
coefficients. The lower limit, r will preserve the
terms of the polynomial, but we need to change
the sign. Altogether, the new polynomial will be
N(r)
|{z}
new polynomial
= P(1)
|{z}
polynomial evaluated at 1
− P(r)
|{z}
original polynomial
5. After that, repeat steps 2. to 4. until the last inte-
gration is completed.
6. Finally, we want to evaluate the function ψ
l,k
(cr)
with fixed positive constant c > 0. Hence, at the
last integration, we consider the evaluation of cr
at the lower limit only.
In practice, when the Wendland functions are
computed, they tend to be presented with integer coef-
ficients, i.e. the integration coefficients must be scaled
by their Least Common Multiplayer (LCM). The co-
efficients in C++ are given as double and to be able
to obtain the LCM one needs integers. Furthermore,
the library to compute the LCM is a Boost library that
requires to clearly define the type of the variable. So,
the integrate coefficient are stored as a different ar-
ray defined as long long unsigned. Once the LCM
is obtained, the Greatest Common Divisor (GCD) is
also computed to make sure that the factor to make
the coefficients integers is the least.
The code that computes the Wendland functions
is divided in three parts and can be found in the ap-
pendix.
1. The first step computes the coefficients of Pascal’s
Triangle for the degree l. If k = 0, then the Wend-
land function will be given by (1 − cr)
l
for any
given value of c. If k 6= 0 then the c parameter
will be not considered in this iteration.
2. The next step is to keep track of iterations up
to k − 1, in this section of the code the product
and the integration will be carried out. The vec-
tor momentaneo, i.e.,“momentanous” is created to
store the numerators momentanously.
The term that corresponds to the evaluation of the
upper limit 1, equals the sum of all the terms.
Since in a hand-made calculation, it would be nec-
essary to keep track of the denominators as well,
following that logic, the denominator is equal to
the LCM, i.e. the divisors(0)=mcm of all the el-
ements of divisors.
3. The last iteration is the most difficult one. It is
given when the value of the last iteration k occurs
and k 6= 0. It follows the same logic: the first vec-
tor is the product that equals the coefficients of the
last integration but is stored in the previous entry
plus one. Now the integration is considered with
the c parameter, namely integral(i+1)=
pow(c,i+1)*product(i)/(i+1);.
In the next step, the divisors are computed, then
the LCM and finally divisors(0)=mcm; in this
case, the upper limit 1 will be as before, but now
we are required to divide by the corresponding c
n
:
integral(0)-=integral(i)/pow(c,i);
Yet again the corresponding numera-
tor will be numerators(N+2*k-l-2-j)
=round(integral(0)*divisors(0)); where
the function round ensures that the number is
integer. In order to compute the GCD it is
necessary to figure out that all the numerators
are correct. However, if one is not interested in
making the coefficient integers, the C++ function
here presented is able to give the corresponding
Wendland functions for double coefficients. The
option is a boolean variable, if equals true then the
Wendland function is scaled. Notice that when
k = 0 the c parameter is computed automatically
according to their value.
Furthermore, when one is interested in using a
non-integer c, one can just activate this options
with a second boolean variable that considers c as
integers only when it is true. This is because when
scaling the polynomial coefficients, one should be
carefully to scale them at the proper time.
Wendland Functions - A C++ Code to Compute Them
325