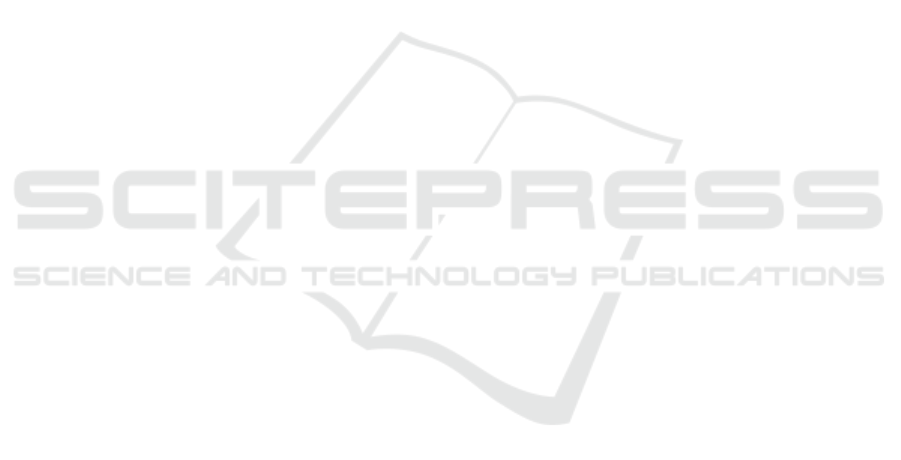
library has backwards compatibility, new versions can
be deployed on the Play Store and automatically up-
dated via the Play Store update protocol. The Google
Play Store could also provide support for live updates
by simply having Amniote reload any classes imple-
mented by the new library, which would be immedi-
ately reflected in individual apps. Linking libraries
through the Google Play Store has the added bene-
fit of security. Since all applications now utilize the
same library version, it is easier to ensure the library
is directly from the vendor and has not been tampered.
10 CONCLUSION AND FUTURE
WORK
In this paper we discussed the growing dependency
of third-party libraries in Android apps. We demon-
strated that many third-party library classes are ref-
erenced by the majority of apps in their specific app
category. We then showed that the probability distri-
bution of third-party classes can be used to construct
an app category profile which statistically informs our
decision to preload a given class.
Next we evaluated the execution of apps in the
Agave benchmark suite on a modified gem5 simu-
lator. The simulations demonstrated that only a rel-
atively small proportion of operations are copy-on-
writes compared to page accesses.
Finally we introduced the Amniote framework, a
user-space interface to the Dalvik VM. Amniote pro-
vides a simple interface for dynamically adding and
removing a variety of image files to the shared boot
classpath, as well as preloading or unloading spe-
cific classes. This framework provides developers and
vendors greater opportunity for fine-tuning a device
based on user preferences.
In the future we plan to extend the Amniote frame-
work with several additional features.
First, we will define a file format for the app cate-
gory profiles and provide direct support for these files.
Then the preloading would be performed automati-
cally by providing the image file and the profile file.
Second, we plan to provide support for resource
files in addition to classes. The naive preloader in the
Dalvik zygote process preloads classes and resource
files, which Amniote can easily extend.
Third, our evaluations used a large, but static
dataset of benchmark apps for selecting preloading
class candidates. In reality, a user may have more
variability in the apps executed such that the user may
run apps across multiple categories in a single session.
In order to target this use case, machine learning could
be utilized to dynamically build the category profiles.
Finally, we plan to develop the Library Play Store
discussed in Section 9. One benefit of Amniote is that
app developers no longer need to focus on third-party
libraries. Library version updates and library version
interdependencies are no longer a concern since the
libraries are stripped from the APK and installed in-
dependently.
The Library Play Store would provide a single
repository for libraries which would additionally add
security and a possibility for live updates. This would
move Android development one step closer to liquid
software deployment, a conceptual goal of continuous
deployment proposed by Gallidabino et. al (Gallid-
abino et al., 2017). Each entity involved in the final
product, the app developers and the library develop-
ers, could independently deploy updates without con-
cerning themselves with the updates of dependencies.
REFERENCES
(2018). F-droid. https://f-droid.org/en/. Online; accessed
30-April-2018.
Android (2018a). Android AppCompat Library V7.
https://mvnrepository.com/artifact/com.android.
support/appcompat-v7. Accessed: 2018-11-26.
Android (2018b). Android Room Compiler.
https://mvnrepository.com/artifact/android.arch.
persistence.room/compiler. Accessed: 2018-11-26.
Android (2018c). Android Room Runtime.
https://mvnrepository.com/artifact/androidx.room/
room-runtime. Accessed: 2018-11-26.
Android (2018d). Android Support Library Annotations.
https://mvnrepository.com/artifact/com.android.
support/support-annotations. Accessed: 2018-11-26.
Android (2018e). Android Support Library V4.
https://mvnrepository.com/artifact/com.android.
support/support-v4. Accessed: 2018-11-26.
Android (2018f). Android Support RecyclerView
V7. https://mvnrepository.com/artifact/com.android.
support/recyclerview-v7. Accessed: 2018-11-26.
Android (2018g). Fragments — Android Developers.
https://developer.android.com/guide/components/
fragments. [Online; accessed 2018-11-26].
AntennaPod (2019). AntennaPod. https://github.com/
AntennaPod/AntennaPod. [Online; accessed 20-Feb-
2019].
Binkert, N., Beckmann, B., Black, G., Reinhardt, S., Saidi,
A., Basu, A., Hestness, J., Hower, D., Krishna, T., Sar-
dashti, S., Sen, R., Sewell, K., Shoaib, M., Vaish, N.,
Hill, M., and Wood, D. (2011). The gem5 simula-
tor. ACM SIGARCH Computer Architecture News,
39(2):1–7.
Brown, M., Yannes, Z., Lustig, M., Sanati, M., McKee,
S., Tyson, G., and Reinhardt, S. (2016). Agave: A
benchmark suite for exploring the complexities of the
android software stack. In Performance Analysis of
ENASE 2019 - 14th International Conference on Evaluation of Novel Approaches to Software Engineering
66