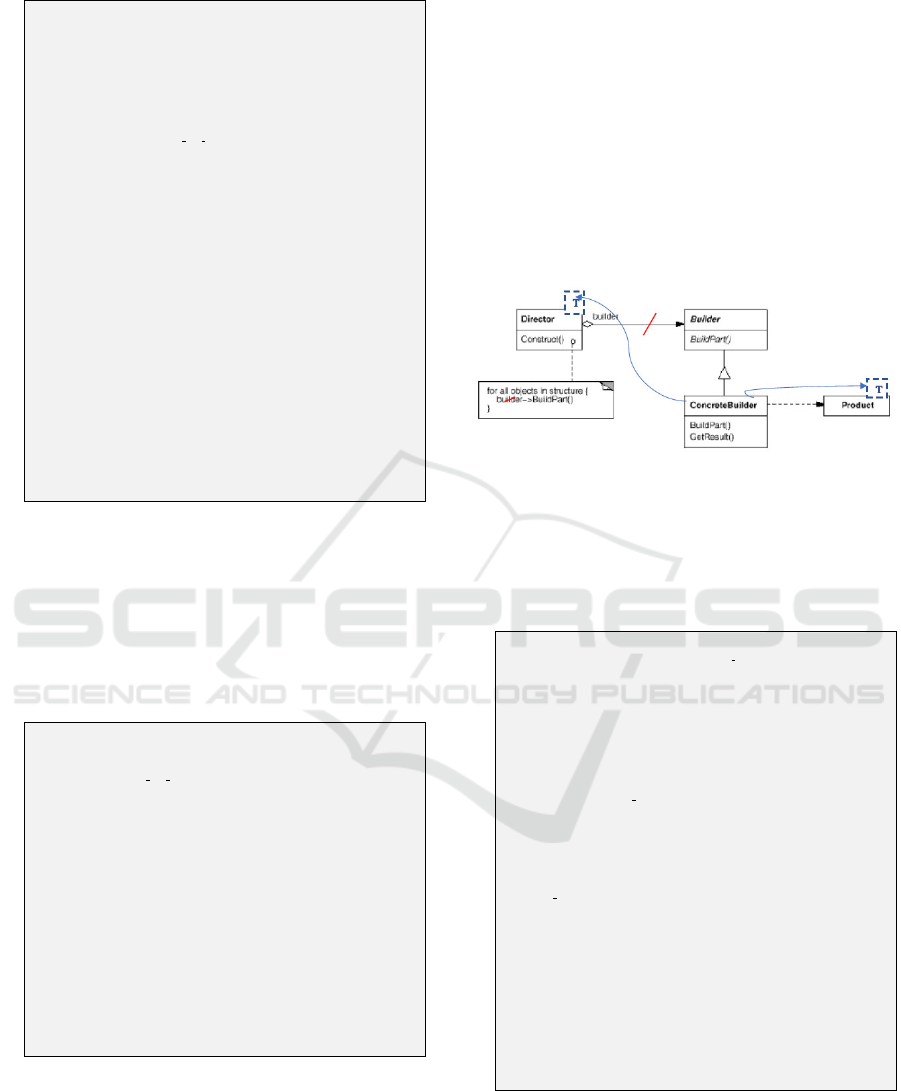
1 t e m p l a t e <t e m p l a t e <c l a s s S > c l a s s T>
2 c l a s s C o l l e a g u e {
3 p r o t e c t e d :
4 S t a t e M e d i a t o r<T>
*
s t a t e ;
5 p u b l i c :
6 C o l l e a g u e ( S t a t e M e d i a t o r<T>
*
s t a t e ) {
7 t h i s −>s t a t e = s t a t e ; }
8 S t a t e M e d i a t o r<T>
*
g e t S t a t e ( ) { r e t u r n s t a t e ; }
9 vo i d c han g e d ( ) {
10 s t a t e −>r e a c t i o n t o c h a n g e ( t h i s ) ; }
11 } ;
12 t e m p l a t e <t e m p l a t e <c l a s s S > c l a s s T>
13 c l a s s C o n c r e t e C o l l e a g u e 1 : p u b l i c C o l l e a g u e<T>{
14 p u b l i c :
15 C o n c r e t e C o l l e a g u e 1 ( S t a t e M e d i a t o r<T>
*
s t a t e )
16 : C o l l e a g u e<T>( s t a t e ) {}
17 vo i d u p d a t e ( ) {
18 / / u p d a t e s o m e t h i n g f o r ' t h i s ' c o l l e a g u e
19 C o l l ea g u e<T> :: ch a ng e d ( ) ; }
20 } ;
21 t e m p l a t e <t e m p l a t e <c l a s s S > c l a s s T>
22 c l a s s S t a t e M e d i a t o r : p u b l i c T<S t a t e M e d i a t o r <T>>
23 { p r o t e c t e d :
24 C o n c r e t e C o l l e a g u e 1
*
c1 ;
25 C o n c r e t e C o l l e a g u e 2
*
c2 ;
26 p u b l i c :
27 v o i d s e t C o n c r e t e C o l l e a g u e 1 (
28 C o n c r e t e C o l l e a g u e 1<T>
*
c ) { v1=c ; }
29 v o i d s e t C o n c r e t e C o l l e a g u e 2 (
30 C o n c r e t e C o l l e a g u e 2<T>
*
c ) { v2=c ; }
31 C o n c r e t e C o l l e a g u e 1<T>
*
g e t C o n c r e t e C o l l e a g u e 1 ( ) {
r e t u r n c1 ; }
32 C o n c r e t e C o l l e a g u e 2<T>
*
g e t C o n c r e t e C o l l e a g u e 2 ( ) {
r e t u r n c2 ; }
33 } ;
Listing 6: Adaptation of the Mediator pattern – Colleague,
ConcreteColleague1, and StateMediator classes.
An object composition is preserved for storing the
set of objects that need to interact; so, the object com-
position is used for an object of type
StateMediator
,
which is used in order to store the team of participants.
The behavior is split out of this class and it is included
through a mixin class – ConcreteMediator.
1 t e m p l a t e <ty pename T>
2 c l a s s Co n c r e t e M e d i a t o r A {
3 p u b l i c :
4 vo i d r e a c t i o n t o c h a n g e ( C o l l e ag u e<Co n c re t eM e di a to r A
>
*
s ) {
5 S t a t e M e d i a t o r <Co n c r e t e M e d i a to r A >
*
s t a t e =
6 s−>g e t S t a t e ( ) ;
7 C o n c r e t e C o l l e a g u e 1<C on c r e t e M e d i a t o r A >
*
c1 =
8 s t a t e −>g e t C o n c r e t e C o l l e a g u e 1 ( ) ;
9 C o n c r e t e C o l l e a g u e 2<C on c r e t e M e d i a t o r A >
*
c2 =
10 s t a t e −>g e t C o n c r e t e C o l l e a g u e 2 ( ) ;
11 / / u p d a t e b a s e d on s p e c i f i c m e d i a t o r s t r a t e g y
12 }
13 } ;
14 / / / / / / / / / us a g e / / / / / / / / /
15 S t a t e M e d i a t o r <Co n c r e t eM e di a to r A> d s ;
16 / / c r e a t e t h e t eam
17 ds . s e t C o n c r e t e C o l l e a g u e 1 ( new Co n c r e t e C o l l e a g u e 1<
C o n c r e t e M e d ia t or A >(&ds ) ;
18 ds . s e t C o n c r e t e C o l l e a g u e 2 ( new Co n c r e t e C o l l e a g u e 2<
C o n c r e t e M e d ia t or A >(&ds ) ;
19 / / u p d a t i n g one d e t e r m i n e a c h a n ge f o r t h e o t h e r s
20 ds . g e t C o n c r e t e C o l l e a g u e 1 ( )−>u p d a t e ( ) ;
Listing 7: Adaptation of the Mediator pattern –
ConcreteMediator class and usage.
If we consider the example given in (Gamma et al.,
1994) with graphic widgets (fields, boxes, ...) this will
translate into a state mediator that store the graphical
objects and several behavior mediators that establish
the particular reaction after a specific widget selection.
Mediator is another example (beside of Decorator)
that combines mixin composition with object compo-
sition: for the state of the team, object composition is
used, but the specific behavior mediator is specified/in-
fused as a mixin.
4.5 Builder
Creational design patterns could be adapted, too.
Builder has a nice solution, in which object composi-
tion between the director and the builder is changed
into a mixin composition.
Figure 5: The adaptation of Builder pattern.
From the Figure 5 it can be seen that the class
Director
is infused with
Builder
specific behavior,
being able to construct, but also to get the result that is
specific to a particular builder – e.g.
ConcreteBuilder
.
Implementation details are give in Listing 8.
1 t e m p l a t e <ty pename T ,
2 typen a m e S = t ypename T : : p a r t t y p e >
3 c l a s s P r o d u c t {
4 S p a r t 1 , p a r t 2 ;
5 p u b l i c :
6 P r o d u c t ( S p1 , S p2 ) {
7 t h i s −>p a r t 1 = p1 ;
8 t h i s −>p a r t 2 = p2 ; }
9 } ;
10 c l a s s C o n c r e t e B u i l d e r {
11 p u b l i c :
12 t y p e d e f i n t p a r t t y p e ;
13 v o i d B u i l d P a r t 1 ( ) { p a r t 1 = 1 0 ; }
14 v o i d B u i l d P a r t 2 ( ) { p a r t 2 = 2 0 ; }
15 Pr o d u c t<C o n c r e t e B u i l d e r > g e t R e s u l t ( ) {
16 r e t u r n P r o d u c t<C o n c r e t e B u i l d e r >(p a r t 1 , p a r t 2 ) ;
17 }
18 p r i v a t e :
19 p a r t t y p e p a r t 1 , p a r t 2 ;
20 } ;
21 t e m p l a t e <ty pename T>
22 c l a s s D i r e c t o r : p u b l i c T{
23 p u b l i c :
24 v o i d c o n s t r u c t ( ) {
25 T : : B u i l d P a r t 1 ( ) ;
26 T : : B u i l d P a r t 2 ( ) ; }
27 Pr o d u c t<T> g e t R e s u l t ( ) { r e t u r n T : : g e t R e s u l t ( ) ; }
28 } ;
29 / / / / / / / / / us a g e / / / / / / / / /
30 D i r e c t o r<Co n c r e t e B u i l d e r > a ;
31 a . c o n s t r u c t ( ) ;
32 P r o d u c t<C o n c r e t e B u i l d e r > pa = a . g e t R e s u l t ( ) ;
Listing 8: Adaptation of Builder pattern.
The link between the builder and its specific prod-
uct is done through the parameter of
Product
class; the
result will have the type
Product<ConcreteBuilder>
.
In this case, the builder specializes also the product.
Though this transformation, the classical object com-
ENASE 2021 - 16th International Conference on Evaluation of Novel Approaches to Software Engineering
266