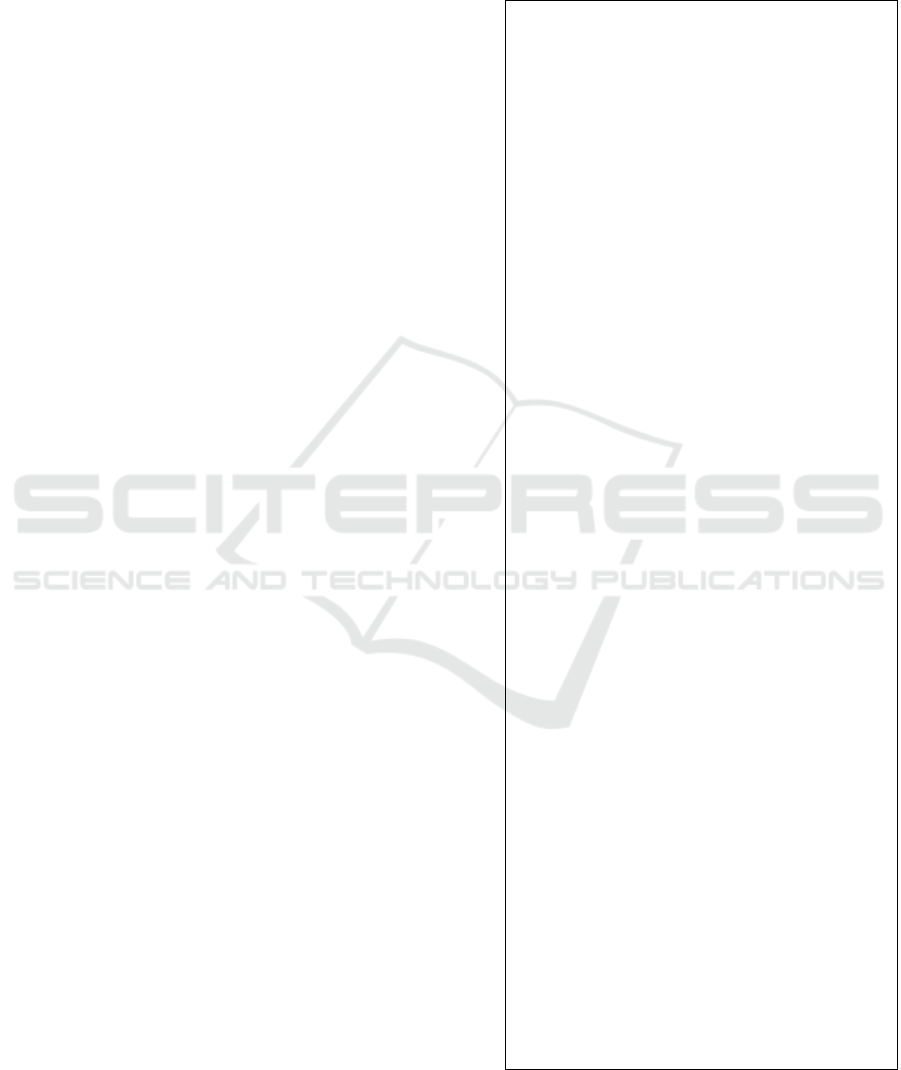
Clarke, E. M., Kroening, D., and Lerda, F. (2004). A tool for
checking ANSI-C programs. In Tools and Algorithms
for the Construction and Analysis of Systems (TACAS).
Springer.
Demmler, D., Dessouky, G., Koushanfar, F., Sadeghi, A.,
Schneider, T., and Zeitouni, S. (2015a). Automated
synthesis of optimized circuits for secure computation.
In CCS. ACM.
Demmler, D., Schneider, T., and Zohner, M. (2015b). ABY -
A framework for efficient mixed-protocol secure two-
party computation. In NDSS. The Internet Society.
Goldreich, O., Micali, S., and Wigderson, A. (1987). How
to play any mental game or A completeness theorem
for protocols with honest majority. In STOC. ACM.
Hastings, M., Hemenway, B., Noble, D., and Zdancewic, S.
(2019). SoK: General purpose compilers for secure
multi-party computation. In S&P. IEEE.
Henecka, W., Kögl, S., Sadeghi, A., Schneider, T., and
Wehrenberg, I. (2010). TASTY: Tool for automating
secure two-party computations. In CCS. ACM.
Holzer, A., Franz, M., Katzenbeisser, S., and Veith, H.
(2012). Secure two-party computations in ANSI C.
In CCS. ACM.
Ion, M., Kreuter, B., Nergiz, A. E., Patel, S., Saxena, S., Seth,
K., Raykova, M., Shanahan, D., and Yung, M. (2020).
On deploying secure computing: Private intersection-
sum-with-cardinality. In EuroS&P. IEEE.
Ishaq, M., Milanova, A. L., and Zikas, V. (2019). Efficient
MPC via program analysis: A framework for efficient
optimal mixing. In CCS. ACM.
Lattner, C. and Adve, V. S. (2004). LLVM: A compilation
framework for lifelong program analysis & transforma-
tion. In International Symposium on Code Generation
and Optimization (CGO). IEEE.
Litvak, S., Dor, N., Bodík, R., Rinetzky, N., and Sagiv, M.
(2010). Field-sensitive program dependence analysis.
In FSE. ACM.
Rosetta Code (2020). Find the intersection of
two lines. https://rosettacode.org/wiki/Find_the_
intersection_of_two_lines#C.2B.2B.
Songhori, E. M., Hussain, S. U., Sadeghi, A., Schneider,
T., and Koushanfar, F. (2015). TinyGarble: Highly
compressed and scalable sequential garbled circuits. In
S&P. IEEE.
Testa, E., Soeken, M., Amarù, L. G., and Micheli, G. D.
(2019). Reducing the multiplicative complexity in logic
networks for cryptography and security applications.
In Design Automation Conference (DAC). ACM.
Yao, A. C. (1986). How to generate and exchange secrets.
In FOCS. IEEE.
APPENDIX
The line intersection algorithm code used in our evalua-
tion (cf. §5) is based on rosettacode.org (Rosetta Code,
2020). Our slightly modified version (with integer
instead of floating-point arithmetic and inlined deter-
minant calculation to challenge our fine-grained arith-
metic decomposition optimization) is given in Lst. 2.
Listing 2: Line-Line intersection in C. The point (
INT_MAX
,
INT_MAX) is used as a sentinel value for no intersection.
1 typedef struct {
2 int x;
3 int y;
4 } Point;
5
6 typedef struct {
7 Point s;
8 Point e;
9 } Line;
10
11 Point LineLineIntersect(
12 int sx1, int sy1, // Line 1 start
13 int ex1, int ey1, // Line 1 end
14 int sx2, int sy2, // Line 2 start
15 int ex2, int ey2) // Line 2 end
16 {
17 Point result;
18
19 // Determinant(sx1, sy1, ex1, ey1);
20 int detL1 = sx1 ∗ ey1 − sy1 ∗ ex1;
21 int detL2 = sx2 ∗ ey2 − sy2 ∗ ex2;
22
23 int sx1mex1 = sx1 − ex1;
24 int sx2mex2 = sx2 − ex2;
25 int sy1mey1 = sy1 − ey1;
26 int sy2mey2 = sy2 − ey2;
27
28 int x
_
nom = detL1 ∗ sx2mex2
29 − sx1mex1 ∗ detL2;
30 int y
_
nom = detL1 ∗ sy2mey2
31 − sy1mey1 ∗ detL2;
32 int denom = sx1mex1 ∗ sy2mey2
33 − sy1mey1 ∗ sx2mex2;
34
35 if (denom == 0) {
36 result.x = INT
_
MAX;
37 result.y = INT
_
MAX;
38 return result;
39 }
40
41 result.x = x
_
nom / denom;
42 result.y = y
_
nom / denom;
43
44 return result;
45 }
46
47 Point mpc
_
main(Line INPUT
_
A, Line INPUT
_
B) {
48 return LineLineIntersect(
49 INPUT
_
A.s.x, INPUT
_
A.s.y,
50 INPUT
_
A.e.x, INPUT
_
A.e.y,
51 INPUT
_
B.s.x, INPUT
_
B.s.y,
52 INPUT
_
B.e.x, INPUT
_
B.e.y);
53 }
Improved Circuit Compilation for Hybrid MPC via Compiler Intermediate Representation
451