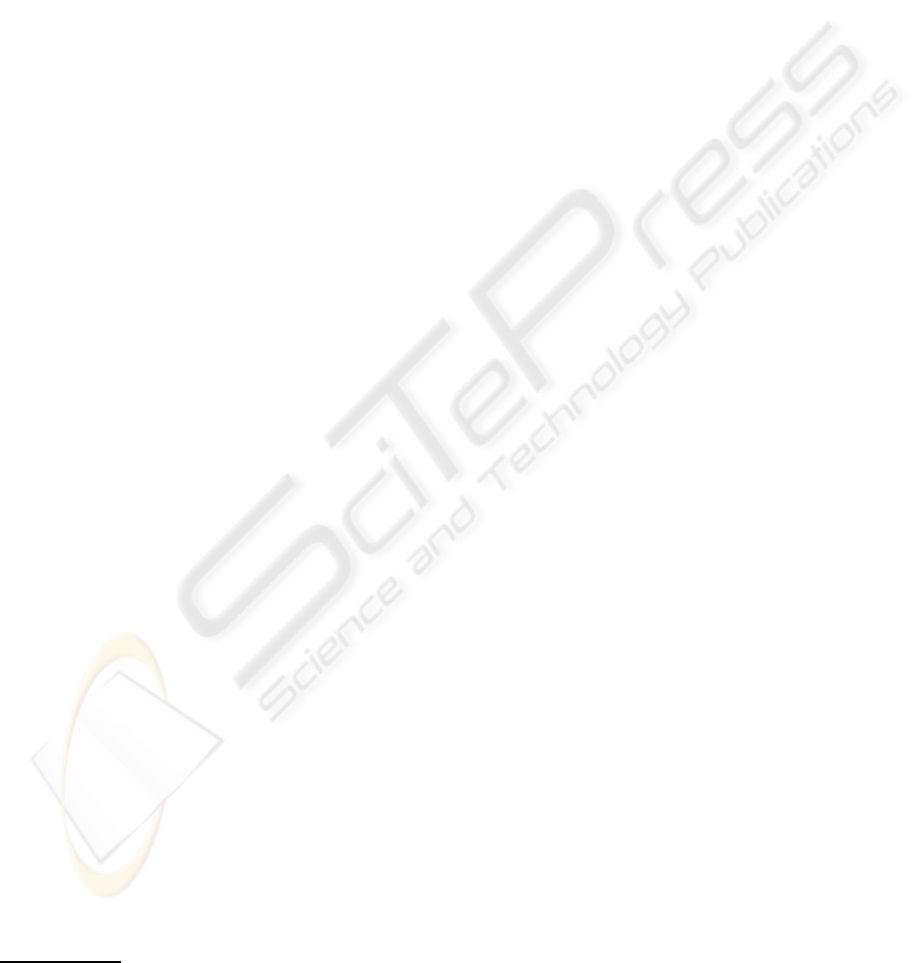
ming style.
To attain these objectives, we compare the ap-
proaches that can be followed in the .NET framework
to access a database and also the methods provided
by .NET along Section 3. To guide this comparison,
a running-example (introduced in Section 2) will be
used. Section 4 closes the paper with some remarks.
2 RUNNING EXAMPLE
The example that we would consider in this paper
is related with an Information System that we de-
velop one year ago (before the latest stable version
of LINQ) in the context of promoting the Portugal’s
northern region.
This information system, called SIGON.2, is
aimed at supporting applicants (private companies or
government institutions) in the the fulfillment of the
application-forms that they should submit to apply for
funds. Under that financial programme, an applica-
tion is eligible if the funds demanded will be used to
promote projects that contribute to the development
of the northern region of Portugal.
Because the application-form is a complex ob-
ject
1
, its fulfillment can not be accomplished at once
(actually this task can last days or even weeks). As
a consequence, it should be possible to perform the
task in several phases. However, no rule should be
imposed, stating where or when these phases start or
end; the applicant should be allowed to interrupt his
work wheneverhe wants, and then continue in another
moment without loosing data.
This statement led us to store (temporarily) the
data fulfilled by each applicant in an intermediate
object, a DataSet, that is then streamed into a line
of a database table, as a XML document. After the
complete fulfillment of the application-form, this data
is convenient stored into the tables of a relational
database. That is the core (the central component) of
SIGON.2.
An application will contain one or more projects
of the same type or of different types. A project can
be one of the type: immaterial (when the contribution
is at the knowledge level); infra-structural (as can be
deduced from the name, this type is concerned with
the development of infra-structures); and mixed (is a
composition of projects that will contribute with both
knowledge improvements and new infra-structures).
Over these undergoing applications, SIGON.2
should be capable of compute some statistics to dis-
1
Composed by a long list of interrelated items that can
vary from a call to another, many of them being dynamic
lists on tables.
play the type of each project. Besides its main func-
tionality, SIGON.2 should be capable of computing
statistics over the undergoing applications (those not
yet closed and submitted, but under fulfillment) by
project type (immaterial, infra-structural, mixed).
To compute these statistics it is necessary to tra-
verse each XML’s DataSet, search for the correct ele-
ment, and count it.
As can be easily deduced, this task requires a con-
siderable amount of time, for the reason that each
XML reflects the structure of a complex application-
form and stores all the fulfilled information.
Because of the great amount of time required to
compute these statistics, we choose this example to
show how it can be implemented using the data source
controls referred previously, as well as, to compare
the performance between them.
3 COMPARING APPROACHES
In this section we compare the three approaches to
access a database, by discussing the resolution of the
practical case-study introduced in Section 2. Besides
the methodological discussion, we will also measure
their performance.
To implement the SIGON.2 statistical feature, as
described in Section 2, all that is necessary is to per-
form a traversal to each XML document (that repre-
sents an application-form)and test if it is of type Infra-
structural, Immaterial or both, to increment the respec-
tive counter.
3.1 LINQ Approach
As the data we want to access is stored in a rela-
tional database, the first thing that we need to pre-
pare before being able to use LINQ with SQL is a data
context class (named
DataContext
). The purpose of
this class is to translate requests for objects into SQL
queries addressed to the database and then assemble
objects out of the results. The data context provides
access to the tables in the database.
Essentially, the data context class maps database
tables into classes, table’s columns in properties, and
relationships between tables are represented by addi-
tional properties.
This class can be automatically generated using
the graphical LINQ to SQL Designer tool (provided
by Microsoft Visual Studio 2008 (Powers and Snell,
2008)) or using the command-line SqlMetal tool (Mi-
crosoft, 2009).
Table 1 displays the code that implements the sta-
tistical feature.
ASSESSING DATABASES IN .NET - Comparing Approaches
279