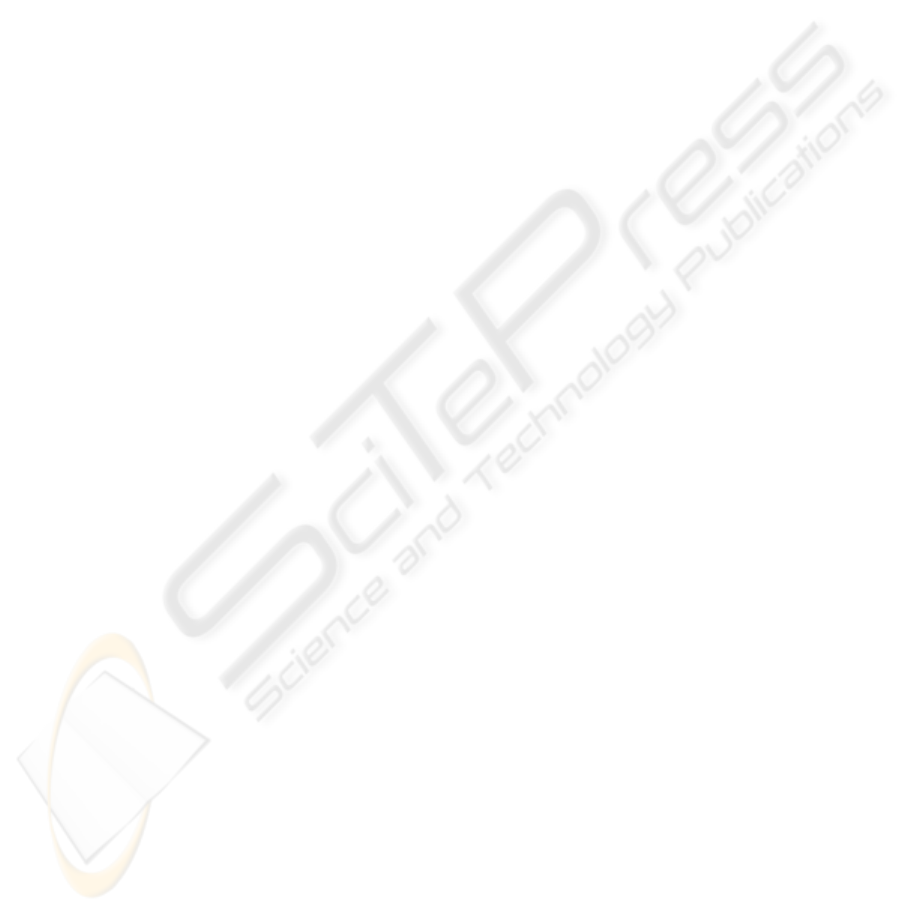
instantiation desired or may set a breakpoint in ev-
ery place the template is instantiated. Another well-
known problem with programming using templates
is that usually the C++ compilers issue quite long
compilation errors in case of problems with template
usage and in particular with template instantiations;
these errors are also hard to understand due to the
presence of parametric types.
Thus, reverse generics can be used as a develop-
ment methodology, not only as a way to turn previous
classes into generic: one can develop, debug and test
a class with all the types instantiated, and then expose
to the “external world” the generic version created
through reverse generics. Provided that an explicit
dependency among reversed generic classes and the
original ones is assumed (e.g., by using makefiles),
the reversed generic version of a class will be auto-
matically kept in sync with the original one.
Classes obtained with reverse generics are not re-
lated to the original classes. We think that this is
the only sensible design choice since generic types
and inheritance are basically two distinguished fea-
tures that should not be mixed; indeed the main design
choices of Java generics tend to couple generics and
class based inheritance (again, for backward compat-
ibility), relying on type erasure, and, as we discussed
throughout the paper, this highly limits the expressiv-
ity and usability of generics in a generic programming
methodology. C++ keeps the two abovefeatures unre-
lated; in particular, the STL library basically does not
rely on inheritance at all (Musser and Stepanov, 1989;
Musser and Saini, 1996; Austern, 1998), leading to a
real usable generic library (not to mention that, avoid-
ing inheritance and virtual methods also leads to an
optimized performance).
We currently described reverse generics in a very
informal way by describing a surface syntax and its
application to Java and C++. We plan to investigate
the applicability of reverse generics also to other pro-
gramming languages with generic programming ca-
pabilities such as, e.g., C# and Eiffel (Meyer, 1992).
As a future work, we will seek a stronger and
deeper theoretical foundation. The starting point
could be Featherweight Java (Igarashi et al., 2001),
a calculus for a subset of Java which was also used
for the formalization of Java generics. Alternatively,
we might use the frameworkof (Siek and Taha, 2006),
which, working on C++ templates that provide many
more features than Java generics, as we saw through-
out the paper, seem to be a better candidate for study-
ing the advanced features of reverse generics.
REFERENCES
Allen, E., Bannet, J., and Cartwright, R. (2003). A First-
Class Approach to Genericity. In Proc. of OOPSLA,
pages 96–114. ACM.
Allen, E. E. and Cartwright, R. (2006). Safe instantiation in
generic java. Sci. Comput. Program., 59(1-2):26–37.
Austern, M. H. (1998). Generic Programming and the STL:
using and extending the C++ Standard Template Li-
brary. Addison-Wesley.
Batov, V. (2004). Java generics and C++ templates. C/C++
Users Journal, 22(7):16–21.
Bracha, G., Odersky, M., Stoutamire, D., and Wadler, P.
(1998). Making the future safe for the past: adding
genericity to the Java programming language. In Proc.
of OOPSLA, pages 183–200. ACM.
Dos Reis, G. and J¨arvi, J. (2005). What is generic program-
ming? In Proc. of LCSD.
Duggan, D. (1999). Modular type-based reverse engineer-
ing of parameterized types in java code. In Proc. of
OOPSLA, pages 97–113. ACM.
Gamma, E., Helm, R., Johnson, R., and Vlissides, J.
(1995). Design Patterns: Elements of Reusable
Object-Oriented Software. Addison Wesley.
Ghosh, D. (2004). Generics in Java and C++: a comparative
model. ACM SIGPLAN Notices, 39(5):40–47.
Igarashi, A. and Nagira, H. (2007). Union Types for Object
Oriented Programming. Journal of Object Technol-
ogy, 6(2):31–52.
Igarashi, A., Pierce, B., and Wadler, P. (2001). Feather-
weight Java: a minimal core calculus for Java and GJ.
ACM TOPLAS, 23(3):396–450.
Kiezun, A., Ernst, M. D., Tip, F., and Fuhrer, R. M. (2007).
Refactoring for parameterizing java classes. In Proc.
of ICSE, pages 437–446. IEEE.
Meyer, B. (1992). Eiffel: The Language. Prentice-Hall.
Moors, A., Piessens, F., and Odersky, M. (2008). Generics
of a higher kind. In Proc. of OOPSLA, pages 423–438.
ACM.
Musser, D. R. and Saini, A. (1996). STL Tutorial and Ref-
erence Guide. Addison Wesley.
Musser, D. R. and Stepanov, A. A. (1989). Generic pro-
gramming. In Gianni, P. P., editor, Proc. of ISSAC,
volume 358 of LNCS, pages 13–25. Springer.
Odersky, M., Spoon, L., and Venners, B. (2008). Program-
ming in Scala. Artima.
Odersky, M. and Wadler, P. (1997). Pizza into Java: Trans-
lating theory into practice. In Proc. of POPL, pages
146–159. ACM.
Siek, J. and Taha, W. (2006). A semantic analysis of C++
templates. In Proc. of ECOOP, volume 4067 of LNCS,
pages 304–327. Springer.
von Dincklage, D. and Diwan, A. (2004). Converting Java
classes to use generics. In Proc. pf OOPSLA, pages
1–14. ACM.
ICSOFT 2009 - 4th International Conference on Software and Data Technologies
46