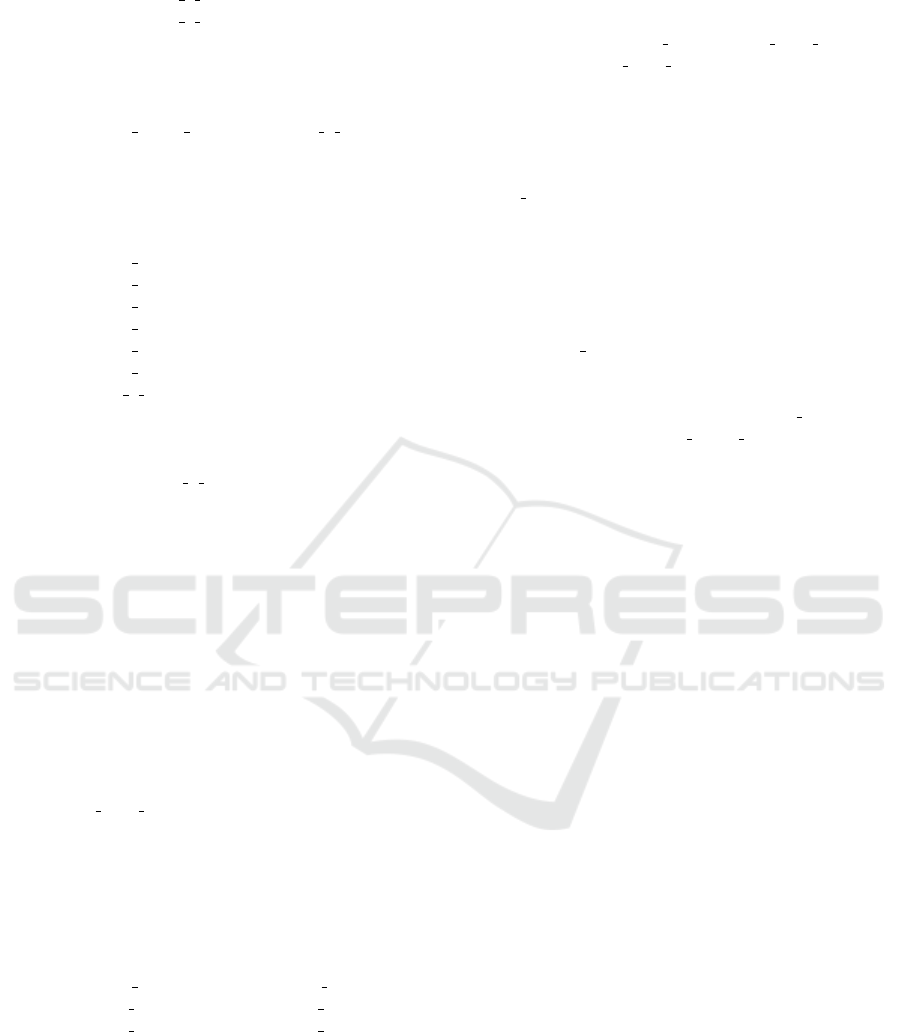
7: edgeList2 0 based.append([i, j])
8: edgeList2 1 based.append([i + 1, j +
1])
9: end if
10: end for
11: end for{Adding the edges:}
12: graphB.add edges from(edgeList2 1 based)
13: c = np.matrix([[0, 1, 0, 0, 0, 0], [1, 0,
1, 0, 0, 0], [0, 1, 0, 1, 0, 0], [0, 0, 1,
0, 0, 0], [0, 0, 1, 0, 0, 0], [0, 1, 0, 0,
0, 0]])
14: graphC = nx.Graph()
15: graphC.add node(1) c1 = Vertex("N", "c1")
16: graphC.add node(2) c2 = Vertex("C", "c2")
17: graphC.add node(3) c3 = Vertex("C", "c3")
18: graphC.add node(4) c4 = Vertex("C", "c4")
19: graphC.add node(5) c5 = Vertex("C", "c5")
20: graphC.add node(6) c6 = Vertex("O", "c6")
21: edgeList3 1 based = []
22: for i in range(0, c.shape[0]) do
23: for j in range(0, c.shape[1]) do
24: if c[i,j] == 1 then
25: edgeList3 1 based.append([i+1,j+1])
26: end if
27: end for
28: end for{In the following part is
attempted the implementation of a QI
Sequence (Lee and collaborators, 2012)
for a graph. } {A data graph from (Lee
and collaborators, 2012):}
{Nodes:}
29: a1 = Vertex("N", "a1"), a2 = Vertex("C",
"a2"),
a3 = Vertex("C", "a3"), a4 = Vertex("C",
"a4"),
a5 = Vertex("C", "a5"), a6 = Vertex("C",
"a6"),
30: graphA node list = [a1, a2, a3, a4, a5,
a6] {Adjacency matrix for an undirected
graph having edge weights:}
31: a = np.matrix([[0, 1.40, 0, 0, 0, 0], [1,
0, 45.10, 0, 0, 5.10], [0, 1, 0, 5.10, 0,
0], [0, 0, 1, 0, 5.10, 0], [0, 0, 0, 1, 0,
5.10], [0, 1, 0, 0, 1, 0]])
32: graphA = nx.Graph()
33: graphA.add node(a1), graphA.add node(a2),
graphA.add node(a3), graphA.add node(a4),
graphA.add node(a5), graphA.add node(a6)
34: edgeList = [(a1, a2), (a2, a1), (a2, a3),
(a2, a6), (a3, a2), (a3, a4), (a4, a3),
(a4, a5), (a5, a4), (a5, a6), (a6, a2),
(a6, a5)]
{Iterating the adjacency matrix:}
35: for i in range(0, a.shape[0]) do
36: for j in range(0, a.shape[1]) do
37: if a[i, j] != 0 and i < j then {i
< j will select the elements above
the main diagonal of the adjacency
matrix}
38: graphA.add
edge(graphA node list[i],
graphA node list[j], weight=a[i,j])
39: end if
40: end for
41: end for
42: P = graphA.edges(data=True)
43: qw = graphA
44: P 2 = []
45: if len(P) > 1 then
46: for i in range(0, len(P)-1) do
47: if qw.degree(P[i][0])
+ qw.degree(P[i][1]) ≤
qw.degree(P[i+1][0]) +
qw.degree(P[i+1][1]) then
48: P 2.append(P[i]) {P[i] is "e"}
49: end if
50: end for
51: i = random.randrange(0, len(P 2))
52: selectedEdgeFromP 2 = P 2[i]
53: end if
In order for a quantum computer to work with the in-
put data, a methodology is needed in order encode it
for the oracle. Thus, an index will have to be assigned
to the query data. For example, a query graph can
have an index 00. Then the oracle will look for this
index by using gates in a quantum circuit that amplify
the amplitude for the outcome 00 (Bick, 2018)(Mc-
Caig, 2021).
The part of the code at lines 1 - 12 represents the cre-
ation of the first graph for the algorithm. The second
graph is created between the lines 13 and 28. The
third graph is created between lines 29 and 41. Let’s
consider that each of these graphs has 6 nodes. Lines
of code from classical computing will be transformed
into quantum computing. The quantum computing
equivalent for this, according to the terminology, is
the following. There are two approaches.
1. There are three input graphs, thus there were cre-
ated three quantum circuits, each graph being en-
coded in a circuit.
2. Let’s consider, for example, three quantum cir-
cuits, each having 6 qubits. Just like it was men-
tioned at the first point, a circuit belongs to a
graph. In this case, each graph is divided in parts
which are assigned to different outcomes. The
qubits interact between each other and exchange
information. From here results that the aforemen-
tioned hypothesis is valid because the nodes of the
graph also interact with each other.
For larger numbers of qubits exist larger numbers of
outcomes. For 6 qubits there are 2
6
= 64 outcomes,
for 15 qubits there are 2
15
= 32768 outcomes and
A Study on Hybrid Classical: Quantum Computing Instructions for a Fragment of the QuickSI Algorithm for Subgraph Isomorphism
507