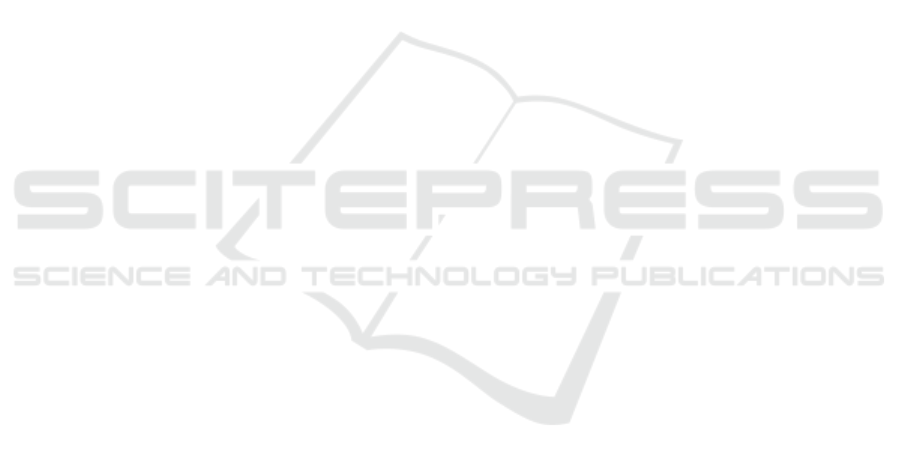
falls short to examine the scenarios in more detail. As
the focus is more on methodology, we only get a ta-
ble that compares the numbers of reversed elements
(theoretical vs. obtained) for the three tools without
a discussion about programming language specific is-
sues and the correctness of the results.
5 CONCLUSIONS
In this paper, we have shown several challenges to re-
verse C++ code to UML. These include language de-
tails as well as the recovery of high-level models. The
latter is currently in general not supported by tools.
In our approach, it only works for a rather small sub-
set of projects: the design recovery from code that
has been previously generated with our approach, as
the implementation patterns are known and carefully
chosen during the design of the code generator with
respect to a bijective mapping.
For the design recovery of legacy code, informa-
tion of applied implementation patterns must be pro-
vided. We hope that deep learning can provide a
means in the future to recover this information, as it
should cope with the fuzzy application of implemen-
tation patterns by developers. In order to train these
mechanisms, we plan to use the code base from the
SW heritage project (Software-Heritage, 2023) and
the fact that a certain subset of the projects contain
both design documents and the associated code allow-
ing us to obtain a training dataset.
ACKNOWLEDGEMENTS
This work has been funded by CEA through the plat-
form Deeplab and by ANR PIA: ANR-20-IDEES-
0002.
REFERENCES
Badreddin, O., Lethbridge, T. C., Forward, A., Elasaar, M.,
and Aljamaan, H. (2014). Enhanced Code Generation
from UML Composite State Machines. Modelsward
2014, pages 1–11.
CDT-developers (2024). Eclipse c/c++ development tools
(cdt). https://projects.eclipse.org/projects/tools.cdt.
Oct. 2023.
Hafeez Osman, M. R. C. (2012). Correctness and Com-
pleteness of CASE Tools in Reverse Engineering
Source Code into UML Model. GSTF Journal on
Computing, 2(1):193–201.
IBM (2023). IBM Rhapsody. https://www.ibm.com/
products/uml-tools. [Online; accessed Oct-2023].
Maro, S., Steghöfer, J.-P., Anjorin, A., Tichy, M., and Gelin,
L. (2015). On Integrating Graphical and Textual Ed-
itors for a UML Profile Based Domain Specific Lan-
guage: An Industrial Experience. In Proceedings of
the 2015 ACM SIGPLAN SLE, pages 1–12. ACM.
OMG (2017). Unified Modeling Language (OMG UML),
Version 2.5.1. OMG Document formal/2017-12-05.
Papyrus-developers (2024). Eclipse Papyrus. https://
eclipse.dev/papyrus/download.html. Oct. 2023.
Pham, V. C., Radermacher, A., Gérard, S., and Li, S.
(2017). Complete code generation from UML state
machine. In Proceedings of the 5th MODELSWARD,
Porto, Portugal, February.
Pham, V. C., Radermacher, A., Gérard, S., and Li, S. (2018).
A New Approach for Reflection of Code Modifica-
tions to Model in Synchronization of Architecture De-
sign Model and Code. In Proceedings of the 6th
MODELSWARD, Funchal, Portugal.
PlantUML (2023). PlantUML website.
https://plantuml.com/. [Online; accessed 10-2023].
Radermacher, A., Cuccuru, A., Gerard, S., and Ter-
rier, F. (2009). Generating Execution Infrastructures
for Component-oriented Specifications With a Model
Driven Toolchain – A case study for MARTE’s GCM
and real-time annotation. In Eighth GPCE’09, pages
127–136. ACM press.
Radermacher, A. et al. (2024). Papyrus Software De-
signer. https://wiki.eclipse.org/Papyrus_Software_
Designer. Oct. 2023.
Rosca, D. and Domingues, L. (2020). A systematic com-
parison of roundtrip software engineering approaches
applied to UML class diagram. In Cruz-Cunha, M. M.
et al., editors, 2020 International Conference on EN-
TERprise Information Systems, volume 181, pages
861 – 868.
Software-Heritage (2023). Software Heritage.
https://www.softwareheritage.org. [Online; ac-
cessed 10-2023].
SparxSystems (2023a). Enterprise Architect.
http://www.sparxsystems.com/products/ea/. [On-
line; accessed Oct-2023].
SparxSystems (2023b). Enterprise Architect, Source
code import. https://sparxsystems.com/enterprise_
architect_user_guide/14.0/model_domains/notes_
on_source_code_import.html. [Online; accessed
Oct-2023].
Stepper, E. et al. (2023). Eclipse CDO Model Repos-
itory. https://projects.eclipse.org/projects/modeling.
emf.cdo. [Online; accessed Oct-2023].
Sutton, A. and Maletic, J. I. (2007). Recovering UML class
models from C++: A detailed explanation. Informa-
tion and Software Technology, 49:212–229.
Tonella, A. and Potrich, A. (2002). Static and Dynamic
C++ Code Analysis for the Recovery of the Object
Diagram. In Proceedings of the International Confer-
ence on Software Maintenance (ICSM’02), Toronto,
Canada. IEEE.
Visual Paradigm (2023). Visual Paradigm Homepage Web-
site. https://www.visual-paradigm.com/. [Online; ac-
cessed 10-2023].
Challenges in Reverse Engineering of C++ to UML
279