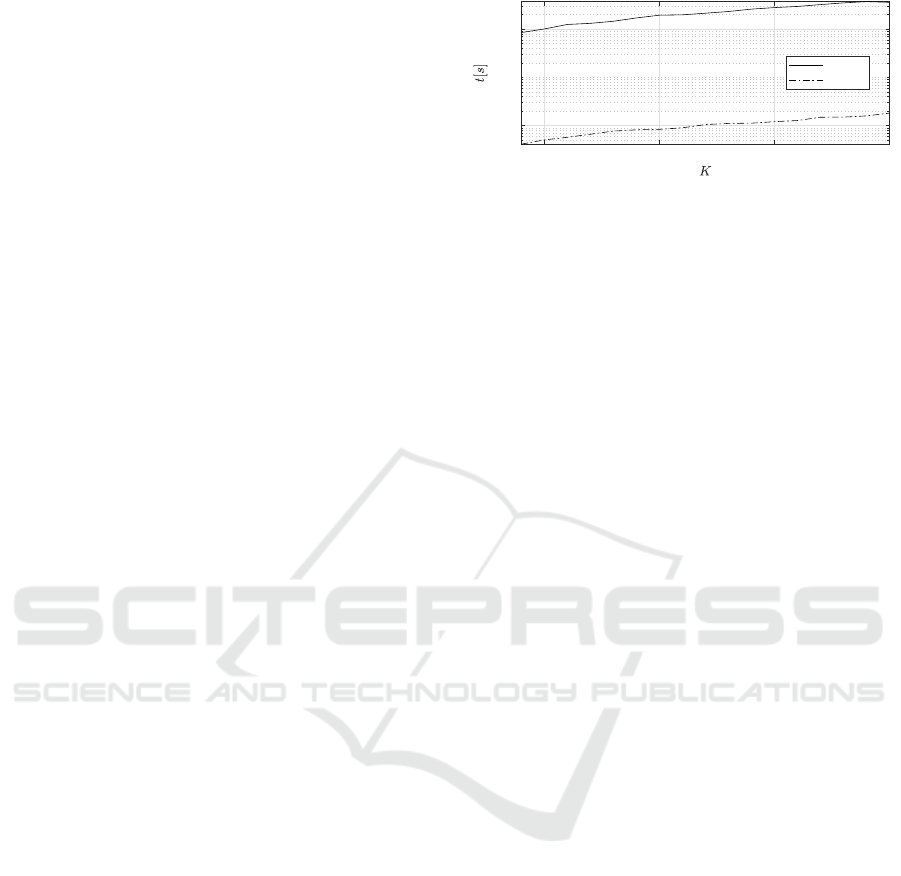
In this way we can define each variable, push into
the Variables vector and then sort it.
All that is left to complete the setup of the LP
problem is defining the constraints. This is were
the benefit of our structure defined in Section 2 is
most apparent since there is no need to keep track of
whether a variable appears twice in a sum, as in (6), or
not. We can simply go through each constraint using
new_aij to add the coefficient of each summand and
close_constr before moving to the next constraint.
As an alternative, we could use a parser such as
YALMIP (L
¨
ofberg, 2004) in MATLAB (The Math-
Works Inc., 2023) to solve for Φ. This would admit-
tedly entail a less convoluted set-up process since we
could create matrix variables and don’t need to imple-
ment the max function with an intermediate variable
like C
ν
i
. However, since MATLAB is an interpreted
language it doesn’t benefit from the efficiency asso-
ciated with compiled languages, in our case the effi-
ciency of the GNU Compiler g++.
For comparison consider the arbitrarily switched
linear system discussed in (Andersen et al., 2023;
Polanski, 1997; Pyatnitskii, 1971; Brockett, 1966)
with subsystems given by
A
1
=
0 1
−0.01 −2
and A
2
=
0 1
−11.7 −2
.
We can compute a CPQ Lyapunov function with a
scaling factor K ≥ 4 and run the computations twenty
times for each K. As can be seen in Figure 3, the av-
erage time for each scaling factor using the C++ im-
plementation is roughly 1/200th of the time needed
when using MATLAB. We used the SDPT3 (Toh
et al., 1999) solver in the MATLAB implementation
and Gurobi version 11 for the C++ implementation.
Additional MATLAB tooling to speed up the compu-
tations like the MATLAB Coder can not be utilized to
minimize computation time without major changes to
YALMIP.
6 CONCLUSIONS
We presented the implementation of a method to com-
pute continuous and piecewise quadratic (CPQ) Lya-
punov functions for switched linear systems in C++;
such a Lyapunov function is a common Lyapunov
function for all the linear subsystems. We compared
our implementation to an analogous implementation
in MATLAB and showed that the C++ implementa-
tion is more than 100 times faster for an example sys-
tem from the literature. We expect this difference in
computational speed to be representative for the gen-
eral case, as the setup of the linear programming (LP)
5 10 15 20
10
-2
10
0
matlab
C++
Figure 3: Comparison of the computational time for param-
eterizing Lyapunov functions with our method in MATLAB
and C++; run on i9900K (8 cores) under Linux Mint. Aver-
age computation time of twenty tests as a function of trian-
gulation scaling factor K in T
K
. Note that the C++ code is
ca. 200 times faster than the MATLAB code.
problem used to parameterize the CPQ Lyapunov
function is quite involved and an interpreted computer
language like MATLAB is at a great disadvantage in
comparison to a compiled language like C++.
REFERENCES
Andersen, S., Giesl, P., and Hafstein, S. (2023). Common
Lyapunov functions for switched linear systems: Lin-
ear programming-based approach. IEEE Control Sys-
tems Letters, 7:901–906.
Baier, R., Gr
¨
une, L., and Hafstein, S. (2012). Linear pro-
gramming based Lyapunov function computation for
differential inclusions. Discrete Contin. Dyn. Syst. Ser.
B, 17(1):33–56.
Brockett, R. (1966). The status of stability theory for de-
terministic systems. IEEE Trans. Automat. Control,
11(3):596–606.
Clarke, F. (1990). Optimization and Nonsmooth Analysis.
Classics in Applied Mathematics. SIAM.
Clarke, F., Ledyaev, Y., and Stern, R. (1998). Asymptotic
stability and smooth Lyapunov functions. J. Differen-
tial Equations, 149:69–114.
Davrazos, G. and Koussoulas, N. (2001). A review of sta-
bility results for switched and hybrid systems. In Pro-
ceedings of 9th Mediterranean Conference on Control
and Automation, Dubrovnik, Croatia.
Dayawansa, W. and Martin, C. (1999). A converse Lya-
punov theorem for a class of dynamical systems which
undergo switching. IEEE Trans. Automat. Control,
(44):751–760.
Deenen, D., Sharif, B., van den Eijnden, S., Nijmeijer, H.,
Heemels, M., and Heertjes, M. (2021). Projection-
based integrators for improved motion control: For-
malization, well-posedness and stability of hybrid
integrator-gain systems. Automatica, 133:109830.
Goebel, R., Hu, T., and Teel, A. (2006). Current Trends
in Nonlinear Systems and Control. Systems and Con-
trol: Foundations & Applications, chapter Dual Ma-
trix Inequalities in Stability and Performance Analy-
sis of Linear Differential/Difference Inclusions, pages
103–122. Birkhauser.
Efficient Implementation of Piecewise Quadratic Lyapunov Function Computations for Switched Linear Systems
283