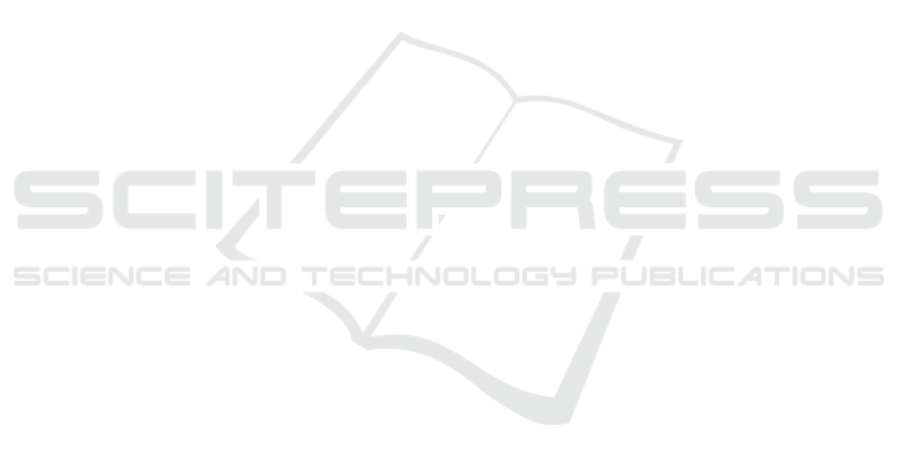
5 CONCLUSION
In this paper, we demonstrated how we can signifi-
cantly speed up the runtime of some GA operators,
including the common bit-flip mutation and uniform
crossover, by observing that such operators define bi-
nomial experiments (i.e., sequence of Bernoulli tri-
als). This enables replacing explicit iteration that gen-
erates a random floating-point value for each bit to
determine whether to flip (for mutation) or exchange
(for crossover), with the generation of a single bino-
mial random variate to determine the number of bits
k, and an efficient sampling algorithm to choose the k
bits to mutate or cross. As a consequence, costly ran-
dom number generation is significantly reduced. A
similar approach is also seen for the generation logic
that determines the number of parents to cross.
The technique is not limited to these opera-
tors, and is applicable for any operator that is con-
trolled by some probability p of including an ele-
ment in the mutation or cross. For example, sev-
eral evolutionary operators for permutations (Ci-
cirello, 2023) operate in this way, including uni-
form order based crossover (Syswerda, 1991), order
crossover 2 (Syswerda, 1991; Starkweather et al.,
1991), uniform partially matched crossover (Cicirello
and Smith, 2000), uniform scramble mutation (Ci-
cirello, 2023), and uniform precedence preservative
crossover (Bierwirth et al., 1996). We adapt this ap-
proach in our implementations of all of these evolu-
tionary permutation operators in the open source li-
brary Chips-n-Salsa (Cicirello, 2020).
REFERENCES
Ackley, D. H. (1985). A connectionist algorithm for genetic
search. In ICGA, pages 121–135.
Baker, J. (1987). Reducing bias and inefficiency in the se-
lection algorithm. In ICGA, pages 14–21.
Bierwirth, C., Mattfeld, D. C., and Kopfer, H. (1996). On
permutation representations for scheduling problems.
In PPSN, pages 310–318.
Cantú-Paz, E. (2002). On random numbers and the per-
formance of genetic algorithms. In GECCO, pages
311–318.
Cicirello, V. A. (2018). Impact of random number genera-
tion on parallel genetic algorithms. In Proceedings of
the Thirty-First International Florida Artificial Intelli-
gence Research Society Conference, pages 2–7. AAAI
Press.
Cicirello, V. A. (2020). Chips-n-Salsa: A java li-
brary of customizable, hybridizable, iterative, parallel,
stochastic, and self-adaptive local search algorithms.
Journal of Open Source Software, 5(52):2448.
Cicirello, V. A. (2022a). Cycle mutation: Evolving per-
mutations via cycle induction. Applied Sciences,
12(11):5506.
Cicirello, V. A. (2022b). ρµ: A java library of randomiza-
tion enhancements and other math utilities. Journal of
Open Source Software, 7(76):4663.
Cicirello, V. A. (2023). A survey and analysis of evo-
lutionary operators for permutations. In 15th Inter-
national Joint Conference on Computational Intelli-
gence, pages 288–299.
Cicirello, V. A. (2024a). Algorithms for generating small
random samples. Software: Practice and Experience,
pages 1–9.
Cicirello, V. A. (2024b). On the average runtime of an open
source binomial random variate generation algorithm.
arXiv preprint arXiv:2403.11018 [cs.DS].
Cicirello, V. A. and Smith, S. F. (2000). Modeling ga per-
formance for control parameter optimization. In Pro-
ceedings of the Genetic and Evolutionary Computa-
tion Conference (GECCO-2000), pages 235–242.
Derrick, B. and White, P. (2016). Why welch’s test is type
I error robust. Quantitative Methods for Psychology,
12(1):30–38.
Flury, B. D. (1990). Acceptance–rejection sampling made
easy. SIAM Review, 32(3):474–476.
Goodman, S. E. and Hedetniemi, S. T. (1977). Introduction
to the Design and Analysis of Algorithms, chapter 6.3
Probabilistic Algorithms, pages 298–316. McGraw-
Hill, New York, NY, USA.
Hinterding, R. (1995). Gaussian mutation and self-adaption
for numeric genetic algorithms. In IEEE CEC, pages
384–389.
Kachitvichyanukul, V. and Schmeiser, B. W. (1988). Bino-
mial random variate generation. CACM, 31(2):216–
222.
Kirkpatrick, S. and Stoll, E. P. (1981). A very fast shift-
register sequence random number generator. Journal
of Computational Physics, 40(2):517–526.
Knuth, D. E. (1998). The Art of Computer Program-
ming, Volume 2, Seminumerical Algorithms. Addison-
Wesley, 3rd edition.
Krömer, P., Platoš, J., and Snášel, V. (2018). Evaluation
of pseudorandom number generators based on residue
arithmetic in differential evolution. In Intelligent Net-
working and Collaborative Systems, pages 336–348.
Krömer, P., Snášel, V., and Zelinka, I. (2013). On the use
of chaos in nature-inspired optimization methods. In
IEEE SMC, pages 1684–1689.
Kuhl, M. E. (2017). History of random variate generation.
In Winter Simulation Conference, pages 231–242.
Larson, H. J. (1982). Introduction to Probability Theory
and Statistical Inference. Wiley, 3rd edition.
Lemire, D. (2019). Fast random integer generation in an in-
terval. ACM Transactions on Modeling and Computer
Simulation, 29(1):3.
Leong, P. H. W., Zhang, G., Lee, D.-U., Luk, W., and Vil-
lasenor, J. (2005). A comment on the implementation
of the ziggurat method. Journal of Statistical Soft-
ware, 12(7):1–4.
ECTA 2024 - 16th International Conference on Evolutionary Computation Theory and Applications
168